Python is an interpreted , object-oriented , high-level programming language with dynamic semantics . It supports module and packages , which encourages program modularity and code re-use.
In python there is no compilation step so the edit-> test -> debug cycle is incredibly fast .
Topics in introduction :
1 : Keywords and Identifiers .
2 : Comments and Indentation .
3: Variables and Data Types.
4 : Standard Input and Output .
5 : Operators .
6 : If -else , while loop , for-loop , break and Continue.
1 : Keywords and Identifiers
Keywords are reserved words in Python , we cannot use them as variable name , function name or any other identifier .
eg :

Identifiers - It is the name given to entities like class, function , variable etc , it helps in differentiating one entity from another.
They can start with (a-z)(A-z)(_ underscore).
Cannot Start with a digit .
Cannot be a keyword.
No Special Characters allowed.
eg :

2 : Comments and Indentation
Comments : They start with # or triple quotes (''' or """) .
DocString (documentation string)
It is a string that occurs as first statement in a module, function , class or method definition .
eg :

To access or see for any function use
>>> print ([function_name].__doc__) ## doc string would be displayed in case it was given during function creation .
Indentation :
Python uses indentation to define a block of code.
Generally 4 spaces is preferred but it can be 8 or more though it should be consistent throughout that block .
eg :
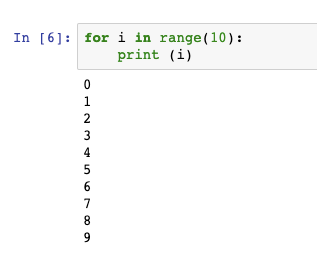
Range Function starts from 0 upto digit (not including last one ie 10) .
Indentation can be ignored in line continuation , but it's a good coding practise to ident always.
eg :
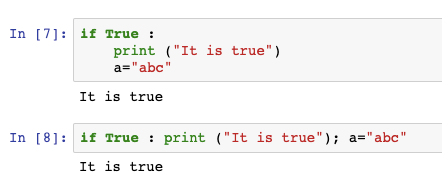
Python Statements : Instructions that a python interpreter can execute are called statements.
eg :
>>> a=1 #single statement
>>> a = 1+2+3+\
4+5+6+\
7+8 # Multi-line statements.
>>> a=(1+2+3+
4+5+6+
7+8)
>> a=10;b=20;c=30
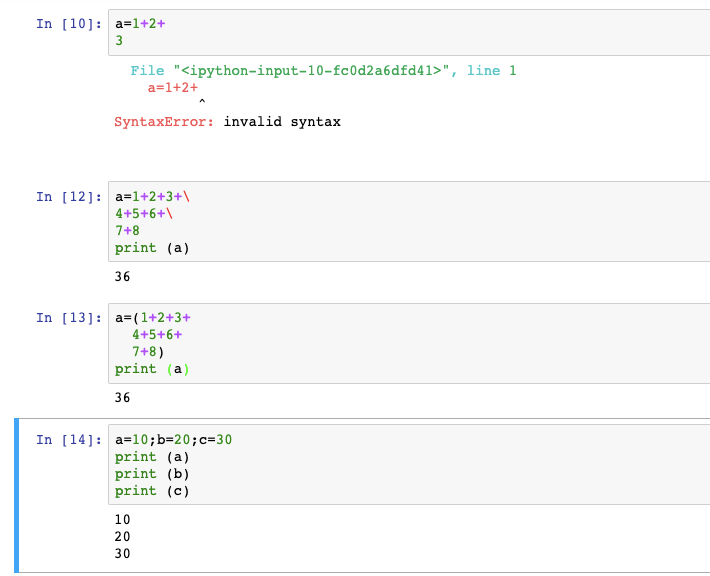
3 : Variables and Data Types
Variables
A Variable is a location of memory used to store some data (value).
Rules of Variables names are similar to identifiers .
We don't need to declare a variable before using it.
We don't declare type of variable , it is handled internally according to type of value we assign to a variable.
eg :
>>> a=10 #int
>>> b=5.5 ## float
>>> c="ABC"
>>> a,b,c=10,5.5,"ABC" ## Multiple assignments is possible in python .
>>> a=b=c="ABC" ## Assigning same value to multiple variables at once can also be done easily .
Storage Location of variables :

both the variables (x and y ) point to same location since both have same value , to efficiently manage memory this is used.
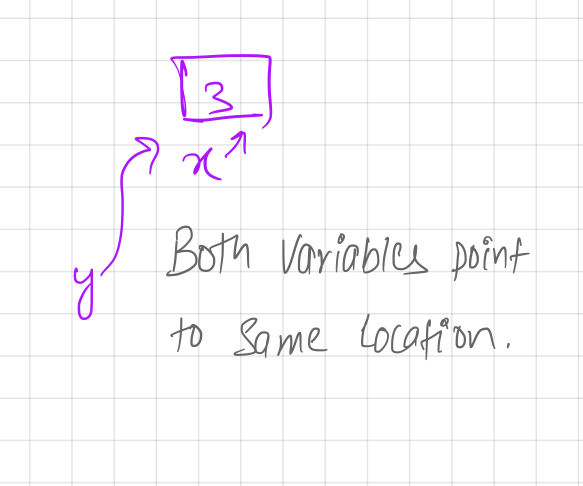
DATA TYPES : Every Value in Python has a datatype .Since everything is an object in python programming , data types are actually classes and variables are instance (object) of these classes.
1 :Numbers
Numbers are of 3 types ie int, float ,complex
use type() to know which class a variable belongs .
eg :

2 : Boolean
Represents truth value False and True .
eg :
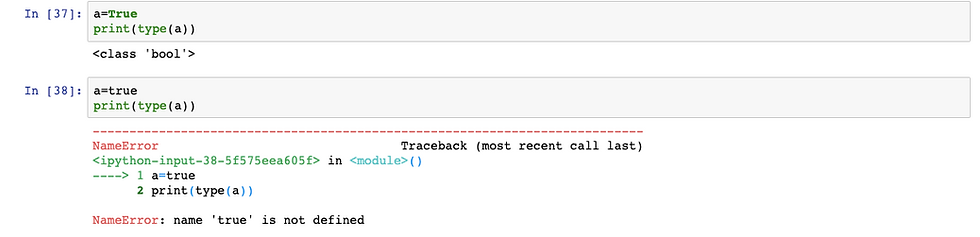
Make sure to use correct syntax ie True and not true or some other declaration .
3: Strings
String is a sequence of unicode characters in Python .
We can use single quotes and double quotes for string . Multi line strings can be represented by tripe quotes (single quotes - ''' and double quotes """.
Strings can be indexed and sliced.
eg :
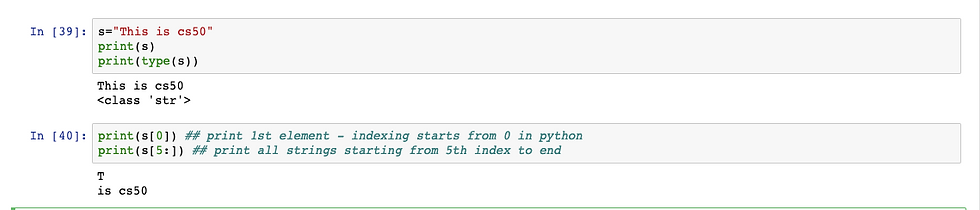
4: Lists
Lists are ordered sequence of items.
All items need not be of same type.
Declared by [] , items separated by , (comma).
Lists are mutable - ie can be altered (elements can be changed/updated).
eg :

5: Tuple
Tuples are ordered sequence of items like list.
They are immutable ie cannot be altered .
Declared by () , items separated by , (comma).
eg :
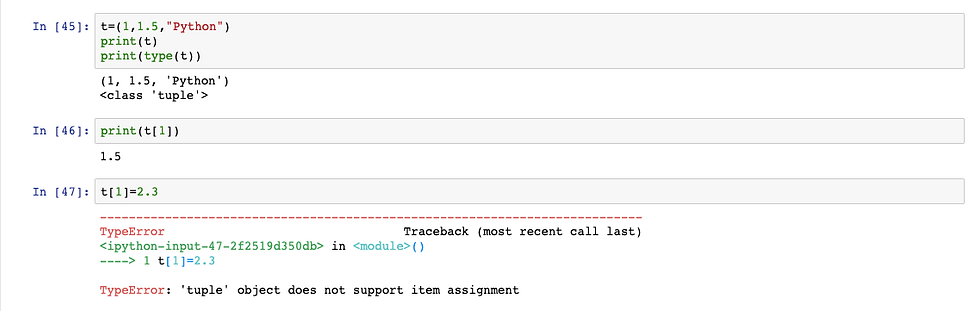
5: Set
It is unordered collection of unique items.
Declared by {} , items separated by comma.
Items in set are not ordered so they cannot be indexed (print(s[1]) ## this cannot be done).
set always have unique value and we can perform - union, intersection etc eg:
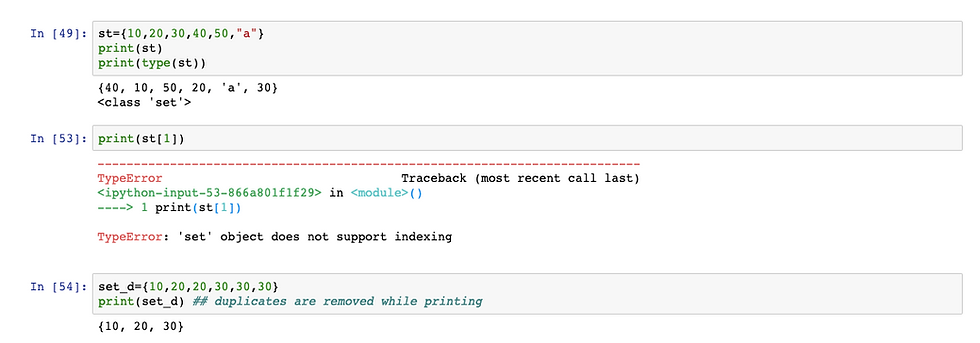
6: Dictionaries
They are unordered collection of Key_value pairs.
Cannot be indexed .
Key-Values are defines in {} , values separated by : and items by , (comma).
key and value can be of any data type.
eg:
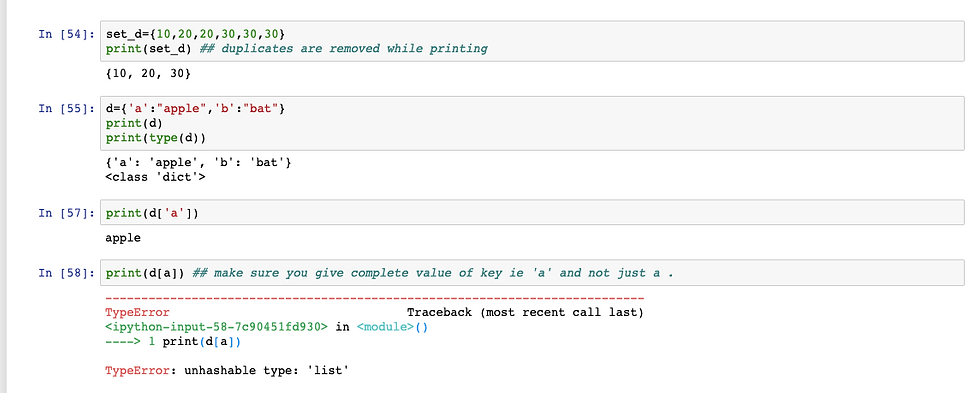
Conversion of Data Types :
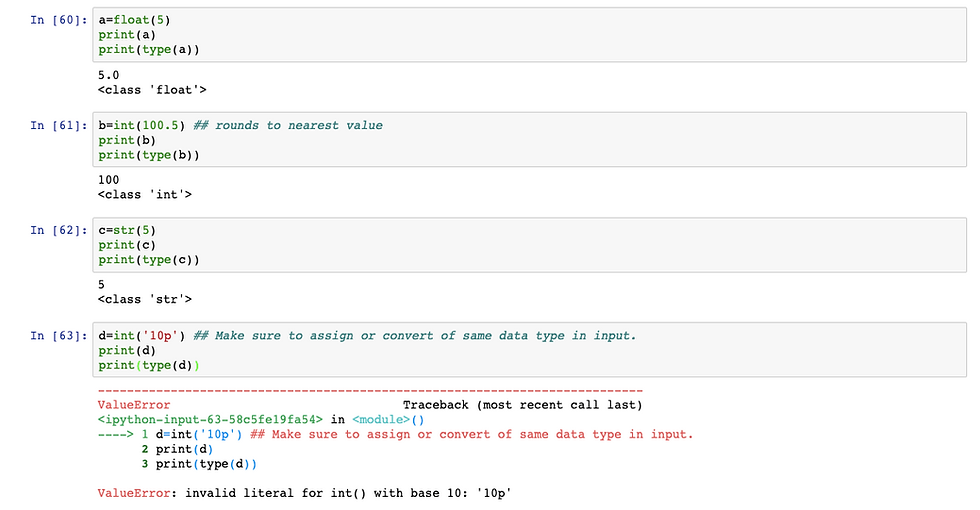
--> We can combine strings using '+' operator (only strings and not int ) .

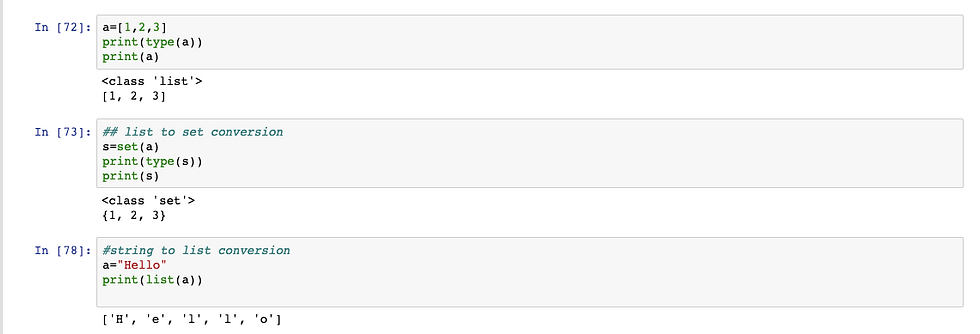
4 : Standard Input and Output
Use print() function to output data to standard output device .
There are many ways through which we can display/use using - + operator, format function, keyword etc .
eg :

5 : Operators
2 + 3 --> + is operator and 2,3 are operands .
Types of operators :
1 Arithmetic : + , - , * , /,% ,// ,**
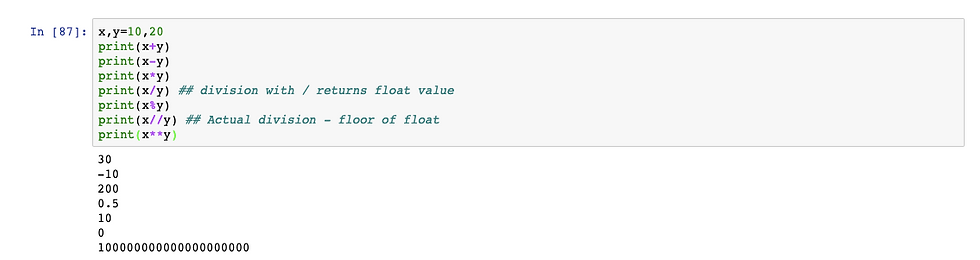
2 Comparison : >,<,==,!=,>=,<=
- They return either True or False .
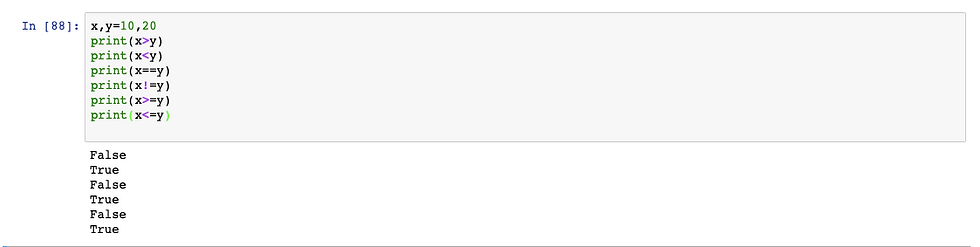
3 Logical : and , or ,not
- They return either True or False as an output .

4 Bit-Wise : &,|,~,^,>>,<<
- They act on operands as if they were string of binary digits . It operates bit by bit.
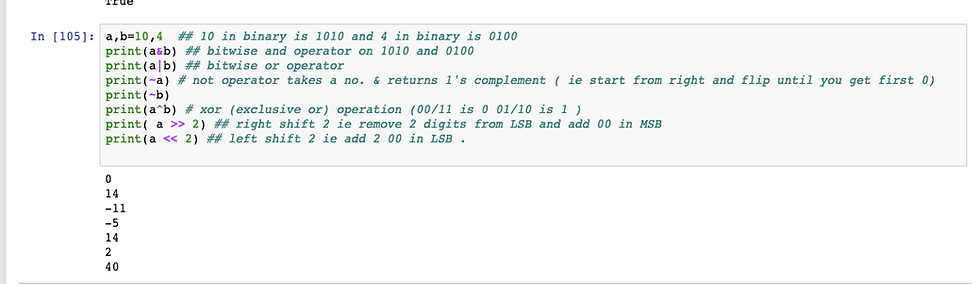
5 Assignment : =,+=,-=,*=,/=,%=,//=,**=,&=,!=,^=,>=,<<=
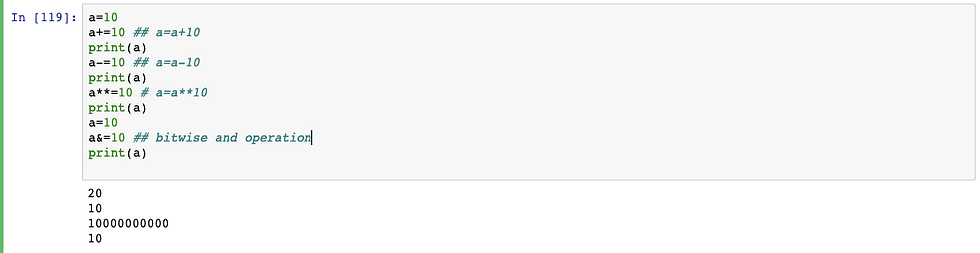
6 Identity : is , is not
- Returns True or False
- They are use to check if two values (or variables) are located on same part of memory or not .
eg :
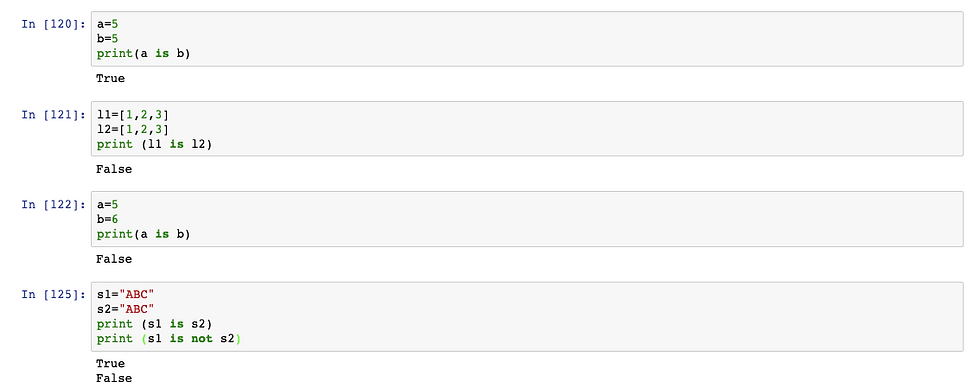
7 Membership : in , not in
- Used to test whether a value or variable is found in a sequence ( string, list, tuple, set and dictionary )
eg :
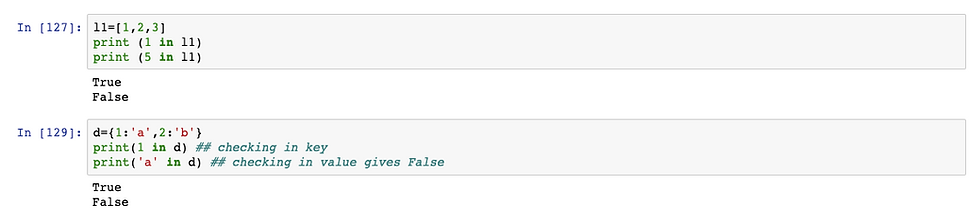
6 : IF-Else and Loops
If-else :
- Python Interprets non-zero as True , None and 0 as False.
eg :
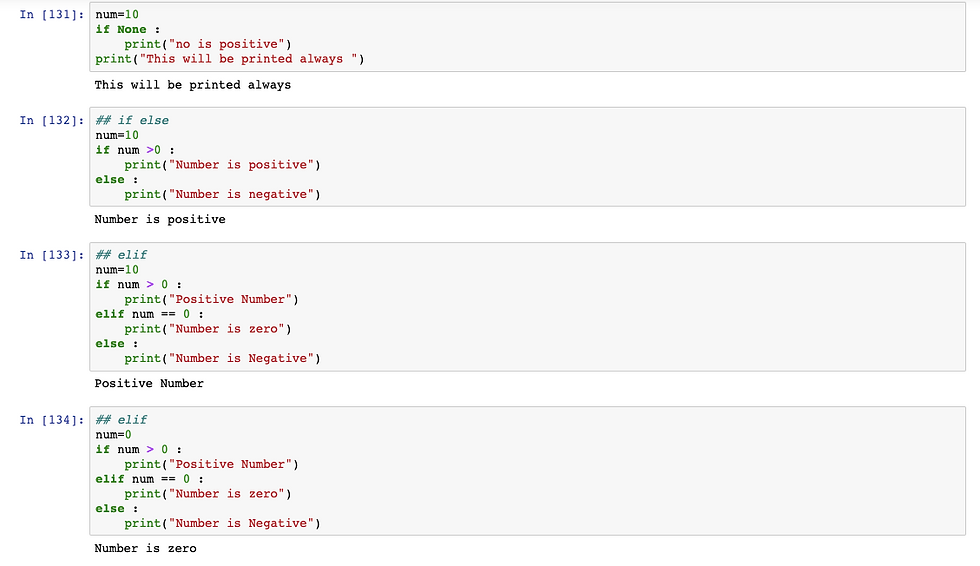
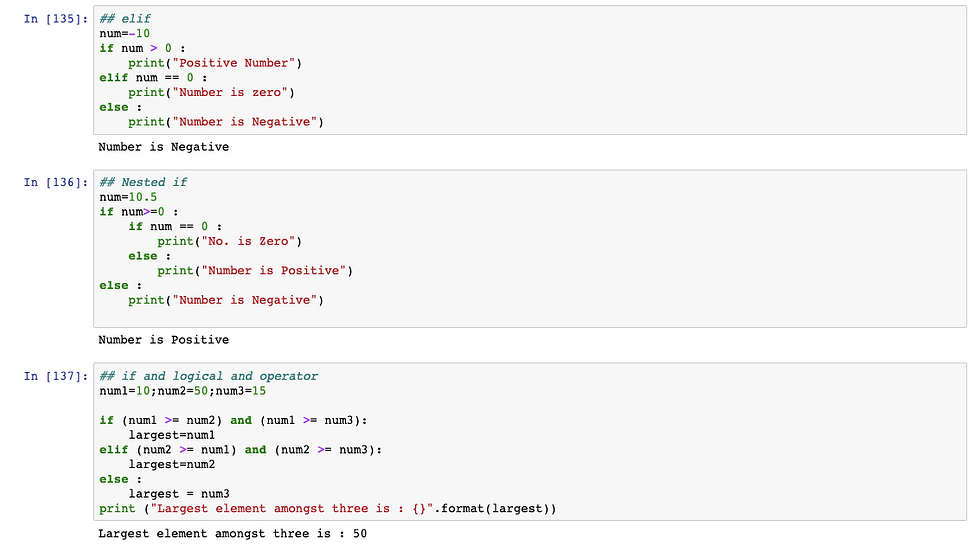
While Loop :
- Loop runs until value becomes false .
- Else in while is evaluated only if condition of while loop evaluates to false .
- While loop can be terminated with a break .
- If there is a break then it does not go into else part .
eg :
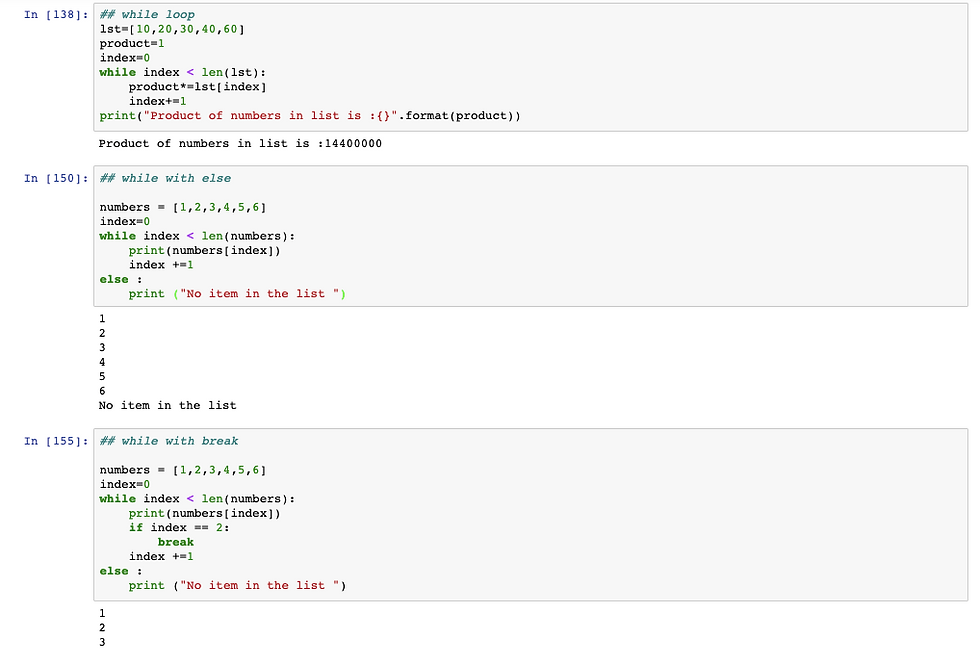
For Loop :
- Loop in Python is used to iterate over a sequence (list, tuple, string) or other iterable objects.
- Loop continues until we reach the last item in the sequence.
- Else part in for loop is executed if the items in the sequence used in for loop exhausts .
- Break statement can be used to stop a for loop and else is also not evaluated.
eg :

Range () Function :
- We can generate a sequence of numbers using range() .
eg : range(10) # it would generate numbers 0 to 9 ie 10 numbers .
- We can also define (start,stop,step_size) in range function # default step size is 1.
- Range function does not store all values in memory just remembers start, stop and step size & generates numbers on the go.
eg :
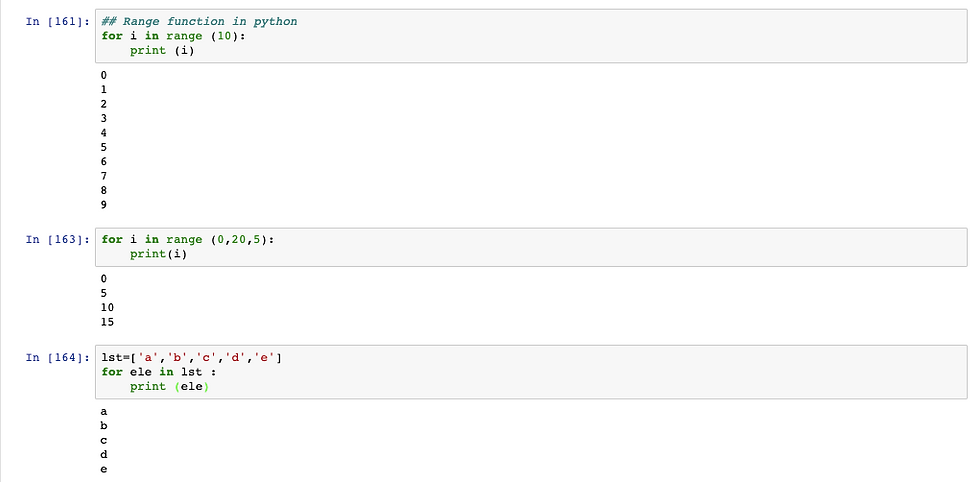
7 : Break and Continue
Both are used to alter the flow of a normal loop .
Break :
In a for loop , in case there is an if condition and then break , so tit comes out of loop.
eg :
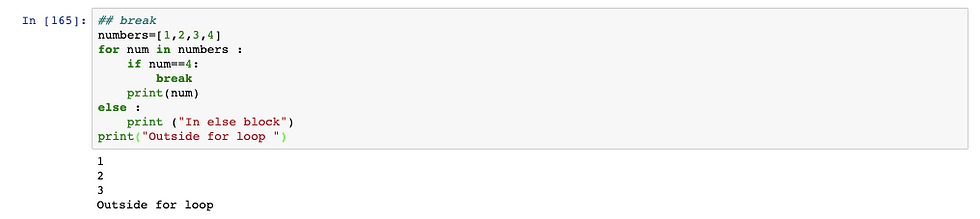
Continue :
- Generally 80% of time is spend in loops .
- Continue takes the flow back to starting of loop without evaluating below code in loop.
eg:
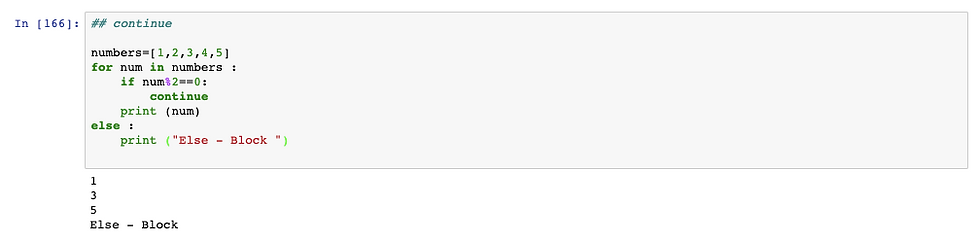
This is a basic introduction to Python ,Hope it helps !
コメント