Function is a group of related statements that perform a specific task.
Functions help break the program into smaller and modular chunks.
Functions avoids repetition and makes code more readable and easy to debug.
def is the keyword used to define a function .
Once defined , a function can be called from anywhere in program .
Return statement
- Used to exit a function and go back to the place where it was called.
- It can contain an expression which gets evaluated and value is returned.
- If no expression in statement then function returns None object .
Scope and Lifetime of variables :
- Variable defined inside a function is not visible from outside.
- Lifetime of a variable inside a function is as long as the function executes.
- Variables are destroyed once we return from the function.
eg :
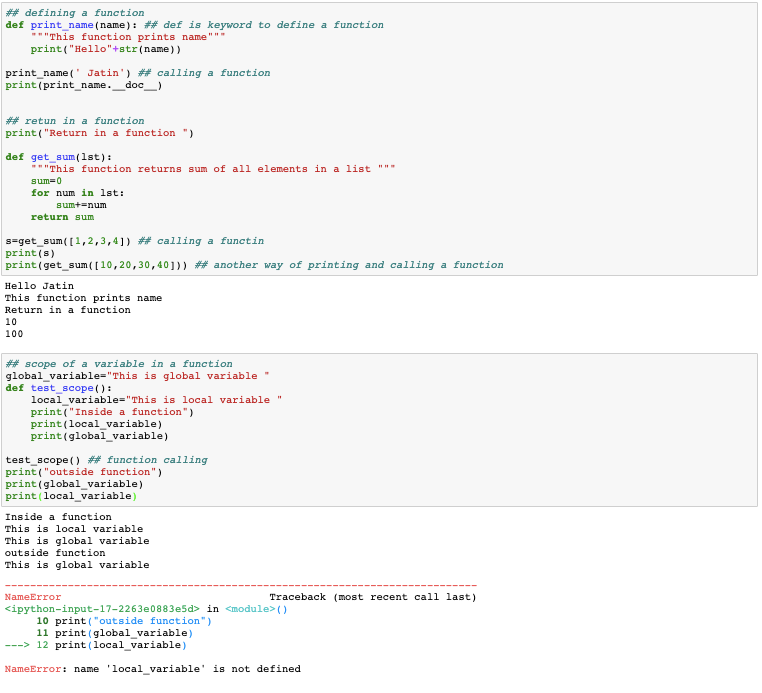
Types of Functions :
1 : Built-In .
2 : User Defined.
1 Built-In :
a : abs() - finds absolute value ie -ve to +ve and +ve to +ve only .
b : all () -
Returns True : If all elements in an iterable (any data structure on which we can iterate over a loop).
False : If any element in iterable is false.
c : dir()
- Tries to return a list of valid attributes of object.
- If object does not have dir() method, this method then tries to find information from dict attribute (if defined) and from type object.
eg :
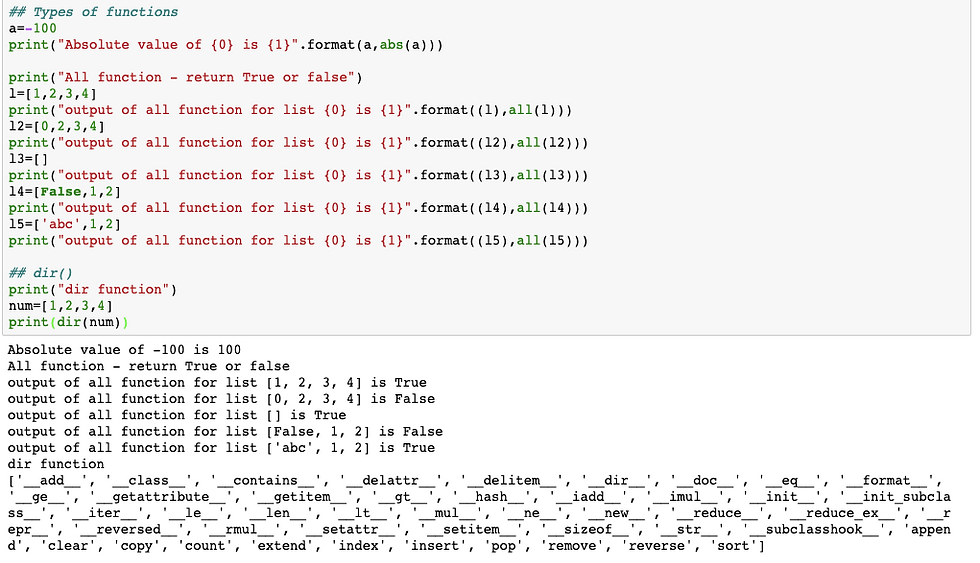
d: divmod()
- Takes 2 numbers and returns a pair of number (a tuple) consisting of their quotient and remainder .
e : enumerate()
- Adds counter to an iterable and returns it .
- Syntax : enumerate(iterable , start=0)
- enumerate in english means to list.
f : isinstance()
- It checks if the object (first argument) is an instance/sub-class of classinfo
class (second argument ) .
- Syntax : isinstance(object , classinfo )
eg :
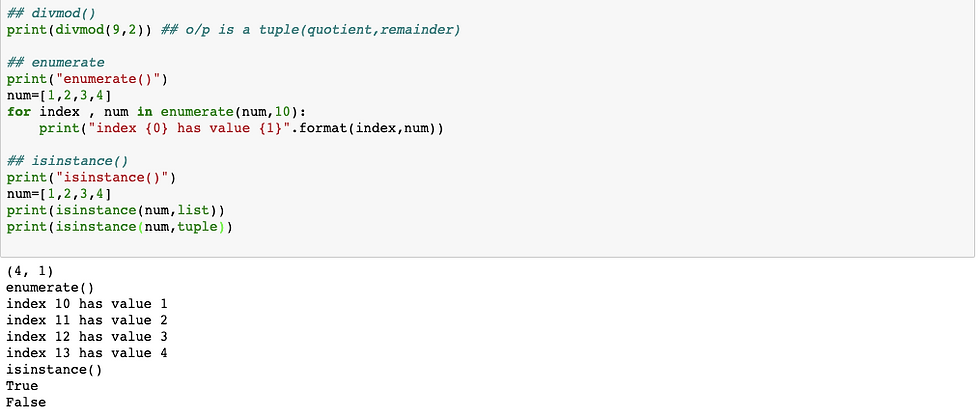
g : filter ()
- Parameters are function and iterable list .
- It constructs an iterator from elements of an iterable for which a function returns true.
- Syntax : filter(function,iterable()).
eg :
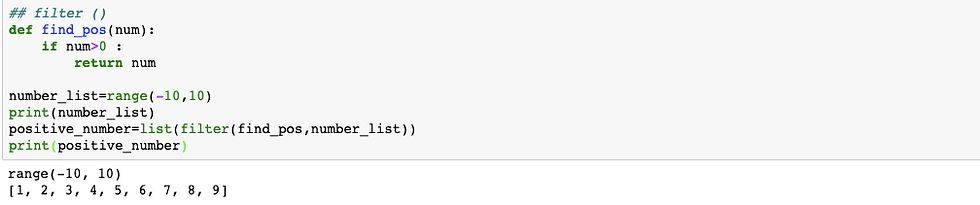
h : map()
- Map applies a function to all items in an inout list .
eg :

i : reduce ()
- It is for performing same computation on a list and returning the result.
- It applies a rolling computation to sequential pairs of values in a list.
eg :
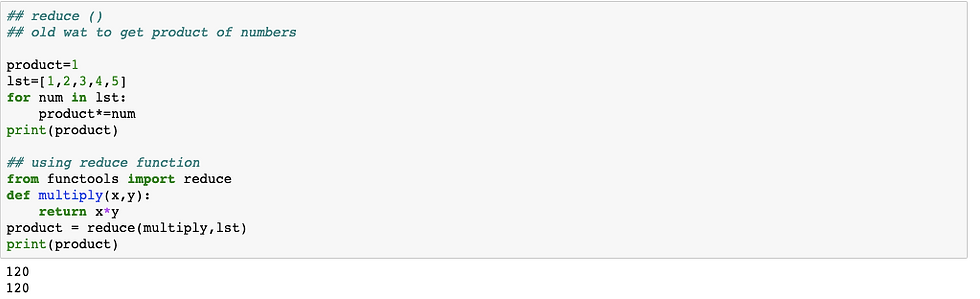
2 User-Defined :
- If we define ourself function to do certain tasks then those are user-defined.
- If we use function written by others in form of library , it can be termed as library functions .
- Helps in decomposing large program into small segments , which makes it easier to understand and debug.
- No repetition of code.
- Devision of workload of programmers.

Function Arguments :
- Arguments are variables/values passed in function .
eg :
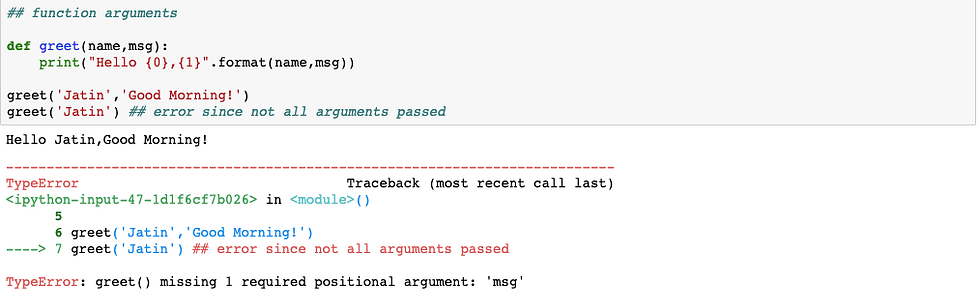
1 - Default Arguments :
- We can pass default value to an argument using (=) operator .
- Once we have default argument then all the arguments to right of it must be default only else error would be displayed.
eg:

2 - Keyword Arguments :
- kwargs allows to pass key-worded variable length of arguments to a function .
- use **kwargs if required to handle named arguments in a function .
eg :
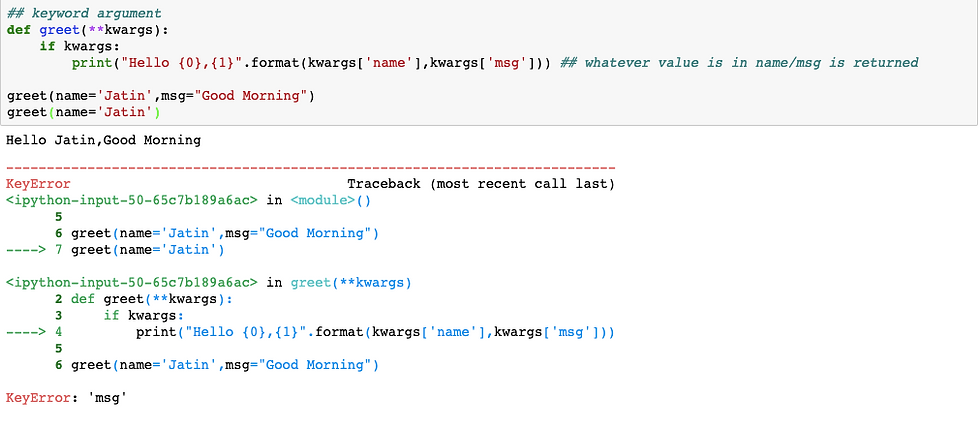
3 - Arbitrary Arguments :
- In case we don't know in advance the number of arguments that will be passed into a function then we use arbitrary arguments.
eg:
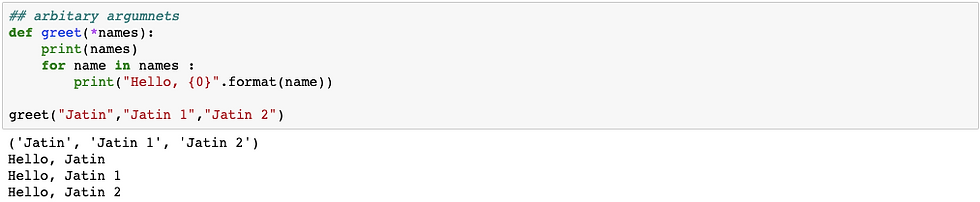
Recursive Functions :
When a function calls itself , it is called as recursive function .
Mostly used in mathematical formulas and data structures.
It makes code look clean and elegant.
Sequence generation is easier with recursion than using nested iterations.
Sometimes logic is hard to follow.
Recursive calls are expensive (inefficient) as they take up a lot of memory and time.
Recursive functions are somewhat hard to debug .
eg:
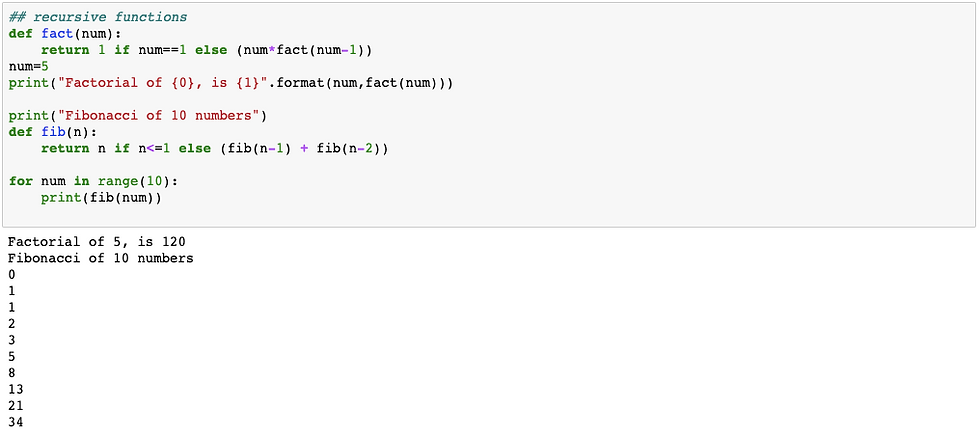
Anonymous/Lambda Functions :
Anonymous function is a function that is defined without a name .
They are defined using lambda keyword , other functions are defined by def keyword.
eg :
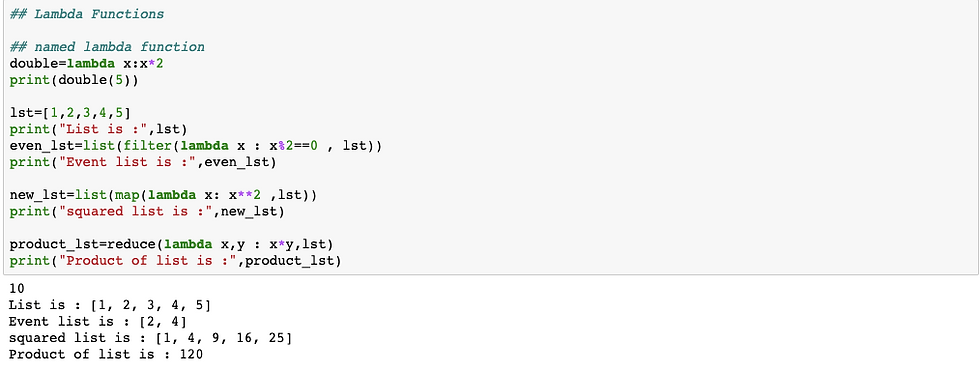
Modules:
Module refers to a file containing python statements and definitions .
A file containing python code eg : abc.py is called a module and its module name is abc.
We use module to break down large programs into small manageable and organised files. Also provides reusability of code.
We can define our most used functions in a module and import it , instead of copying their definition into different programs .
We can also import specific names/functions from a module without importing entire module and all functions.
We use dir() to get find out names that are defined inside a module.
eg:
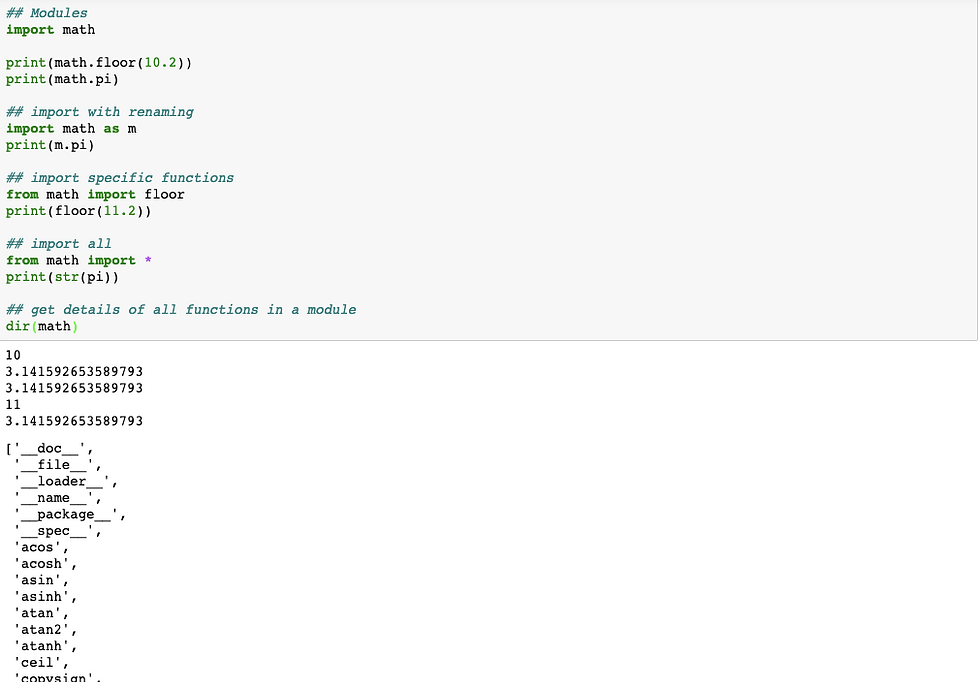
Packages :
Packages are way of structuring python's module namespace by using "dotted module names "
A directory must contain a file name init.py in order for python to consider it as a package . It can be empty or we can place initialisation code .
All package or sub-package must contain init.py file .
Packages are folders for python to consider any folder as a package it must have init.py file .
eg :
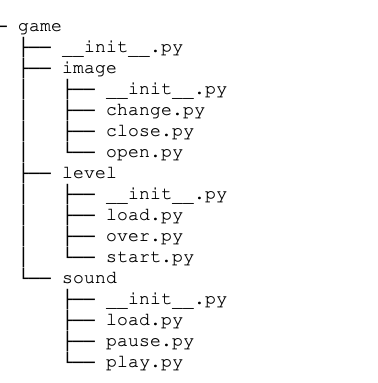
Here game is a package , image , level and sound are sub-packages .
Comments