File Handling
File is a named location on disk to store related information.
Whenever we read/write , first we need to open a file .
Once done , we should close the file so that resources tied to it are released.
Mainly 5 file operations :
Opening a file.
Closing a file.
Writing to a File.
Reading from a file .
File and OS commands .
1. Opening a file :
eg :
>>> f = open('example.txt') ## opens a file in current directory , f is a file handler/pointer.
Modes :
'r' - Opens for reading (default).
'w'- Writing - Creates a new file if it does not exists or truncates if file already exists.
'x' - Opens a file for exclusive creation ie if file exits then operation will fail.
'a' - Opens for appending at end of file, creates a new file if it does not exists.
't' - Opens in text mode (default).
'b' - Opens a file in binary mode.
'+' - Opens a file for updating (reading and writing).
- Default encoding (Python interpreter cannot talk to files directly ie it goes to os which gets file - utf-8 on linux and returns back to python interpreter ) is platform dependent ie windows - 'cp1252' and linux 'utf-8'.
- Always working with text files it is recommended to specify encoding type.
>>> f=open ('example.txt','r',encoding='utf-8')
2. Closing a file :
Frees up resources that were tied with the file.
Close is used so that file can move from RAM to HDD.
Python has garbage collector to clean up unreferenced objects but , we must not rely on it to close the file.
If there is any exception then file may not get closed , so preferred to use with finally (always a cleaner and safer way ).
We can close without close () function .
eg :
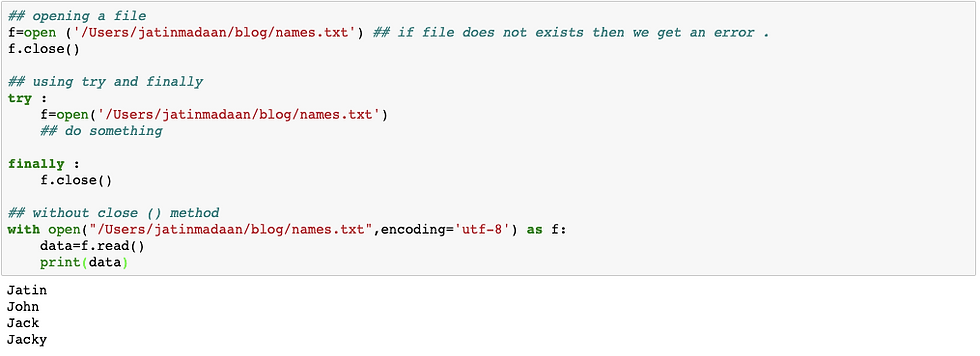
3. Writing to a file :
In order to write to a file we must first open it in write 'w' or append 'a' mode or exclusive creation 'x' mode.
Writing a string or sequence of bytes (for binary files) is done using write() method.This method returns number of characters written to a file.
eg :

4. Reading from a file :
eg :
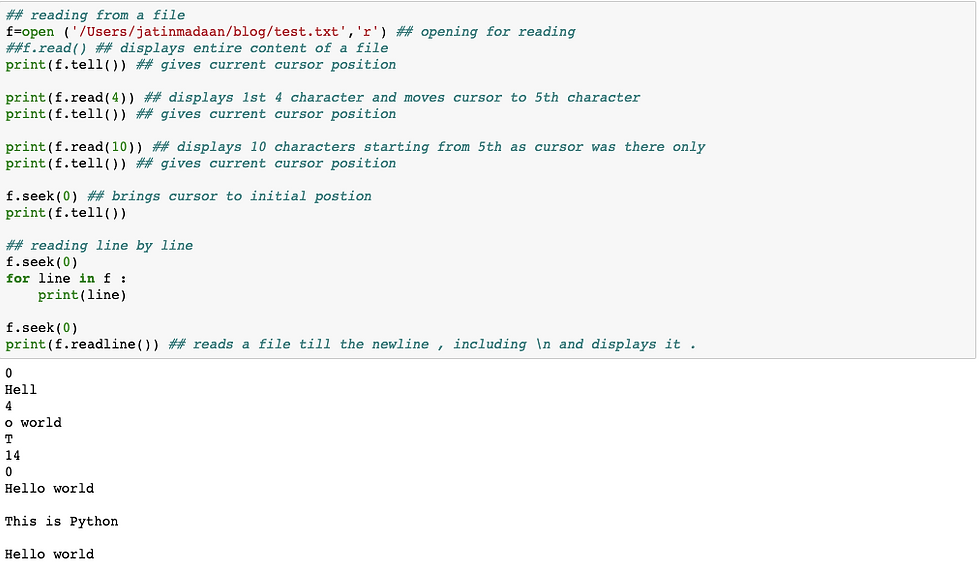
5. File and OS commands :
Renaming and Deleting files :
eg :

Directory and File Management : eg:
eg :
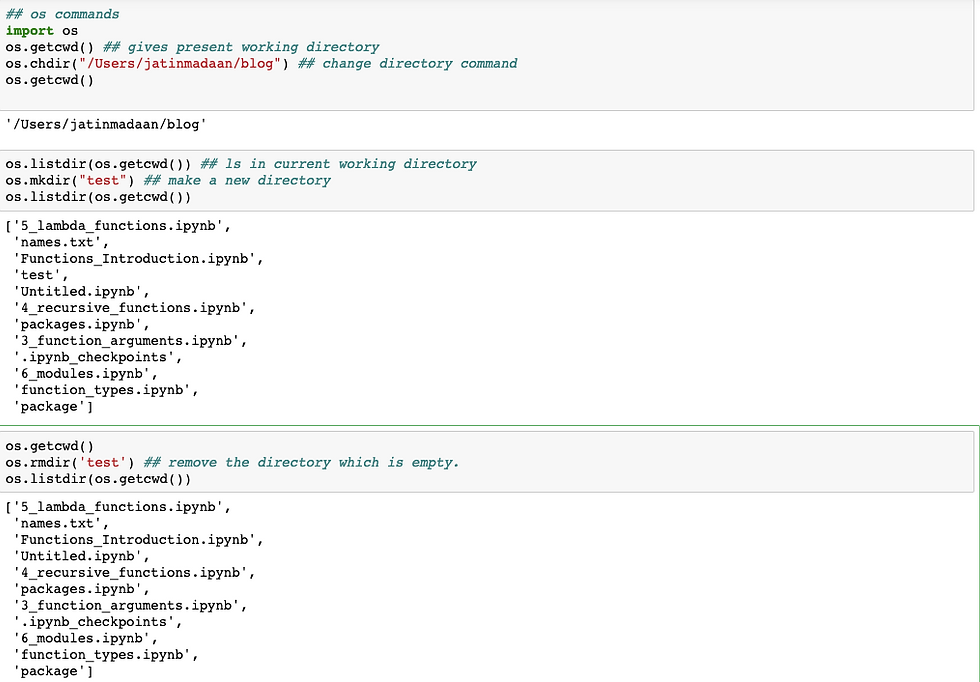
Exceptional Handling
Errors caused by not following proper structure (syntax) of the language are called syntax error or parsing error.
By default as soon as error is raised by python parser program execution stopes there.
eg :

Errors can also occur at runtime and these are called as exceptions .
eg :

All types of exceptions which python handles can be viewed by dir(__builtins__)
eg:
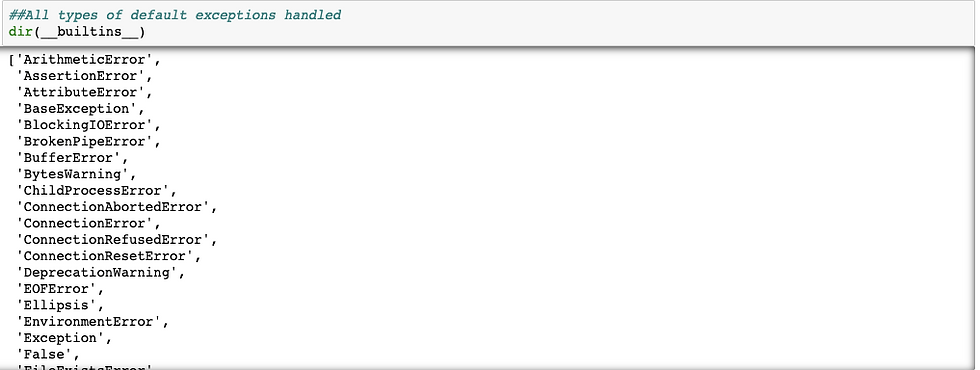
Try ,Except and Finally :
- If no error then except block is not executed.
eg :
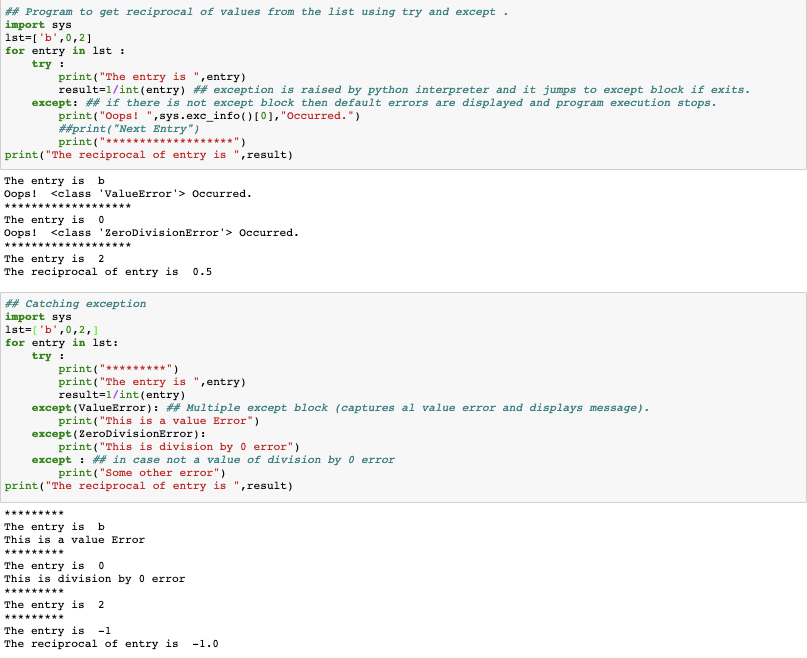
- We can forcefully raise exceptions .
eg :

- No matter what is executed finally always gets executed, generally used to release resources.
eg :

Debugging
pdb(python debugger) implements an interactive debugging environment for python programs .
Starting debugger :
eg:
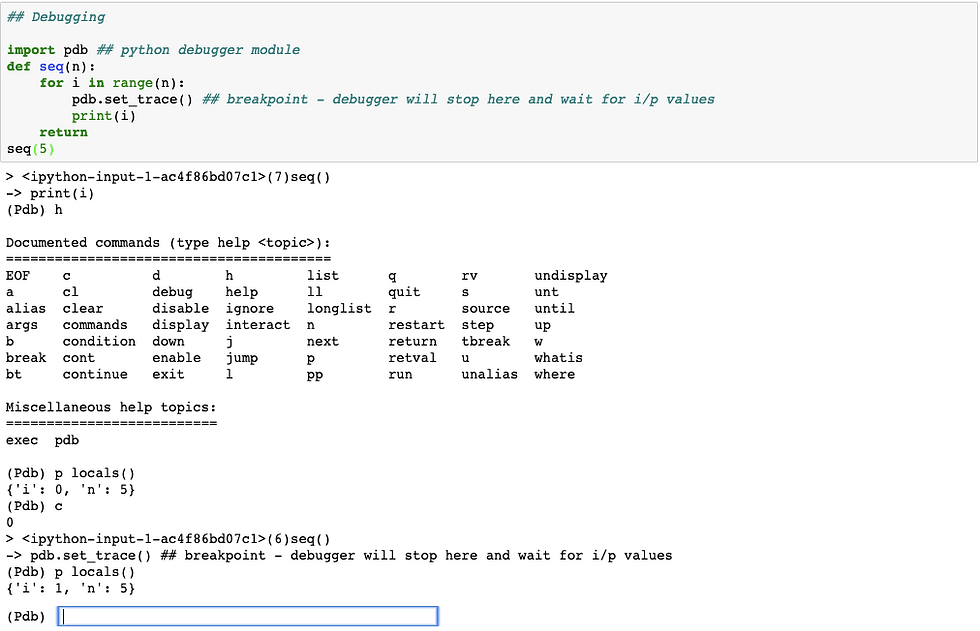
I/p values :
- c : Continue.
- q : Quit .
- h: Help.
- list : Lists where exactly we are.
- p: Print
- p locals() : Prints local variables value.
- p globals() : Prints all global variables .
- h: Here - Prints a stack trace.
- d : Down[count] - moves the current count (default 1) level down in stack trace.
Comments