Data structure is a collection of data elements (such as numbers or characters or even other data structures) that is structured in some way eg : numbering the elements .
Types of Data Structures :
1 - Lists :
List is sequence data structure.
It is Collection of items ie Strings , int or other lists .
Enclosed in [] (square brackets).
Each item has assigned index value.
Each item is separated by comma.
Lists are mutable ie values can be changed.
List operations :
a - List creation :
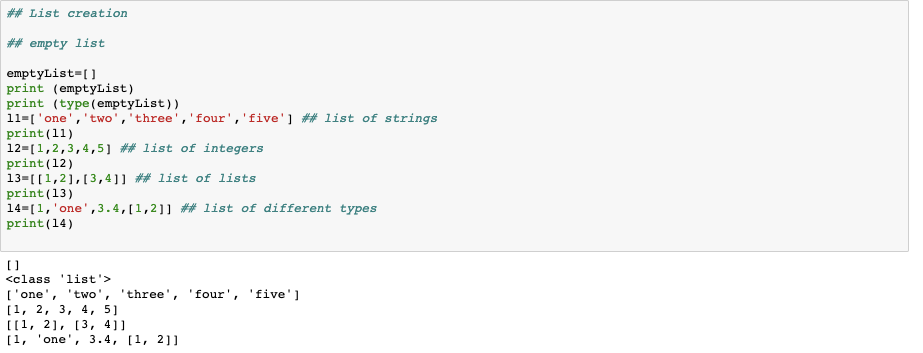
b - List length :

c - List Append :

d - List Insert :
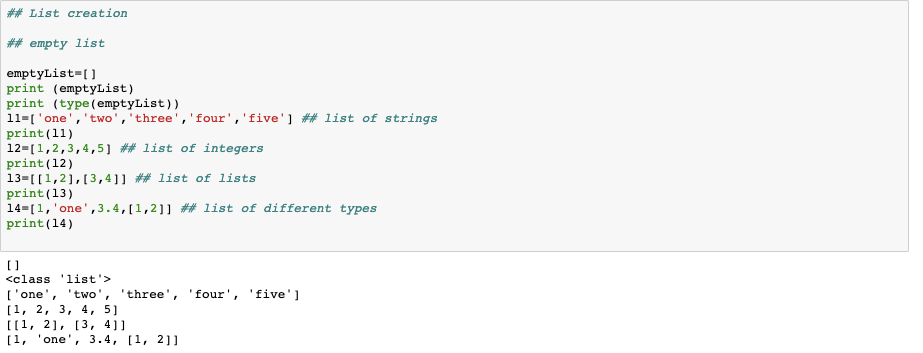
e - List Remove :

f - List Extend :

g - List Delete :
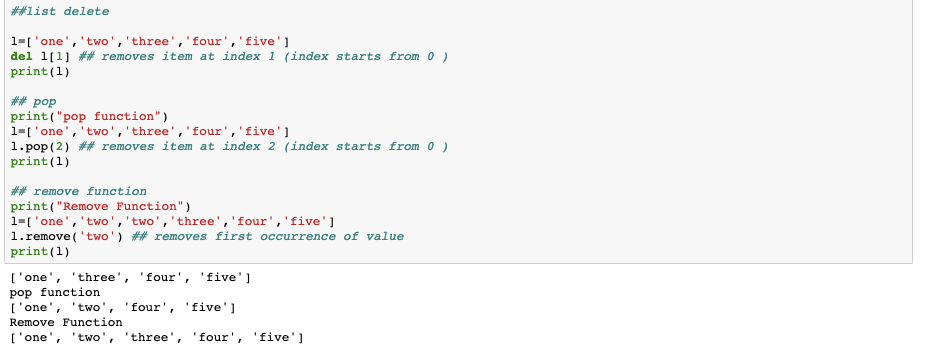
h - List Keywords :
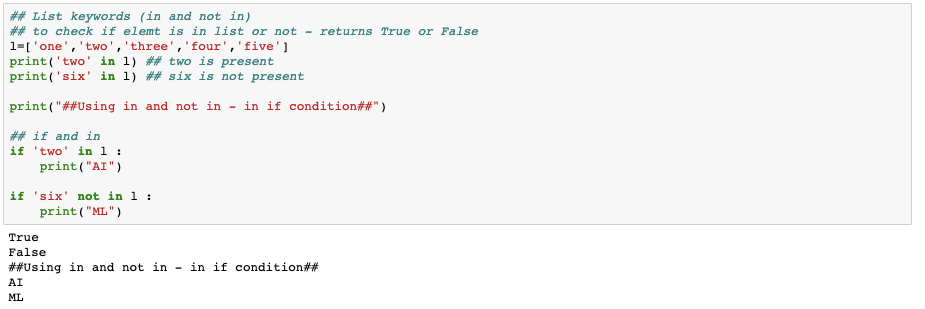
i - List Reverse :

j - List Sorting :
- Returns a new list in sorted order.
- Original list not changed.
- Optional argument (reverse=True).
- In-place sorting can also be done.

k - List with multiple references :
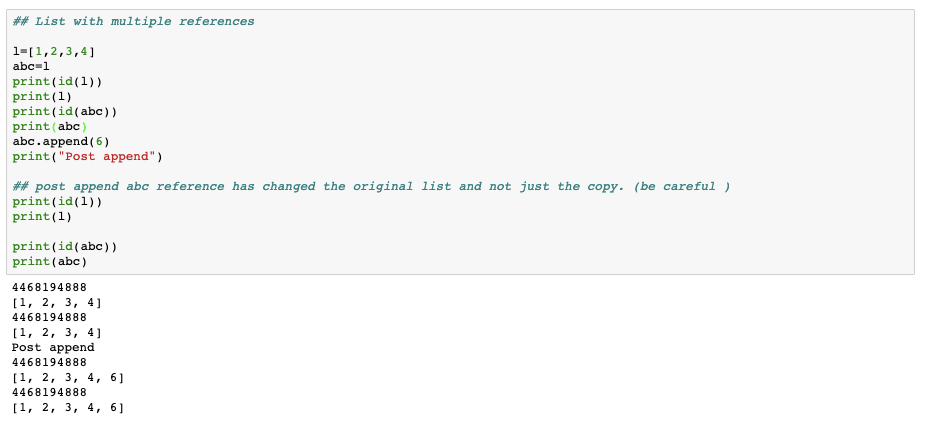
l - String split :
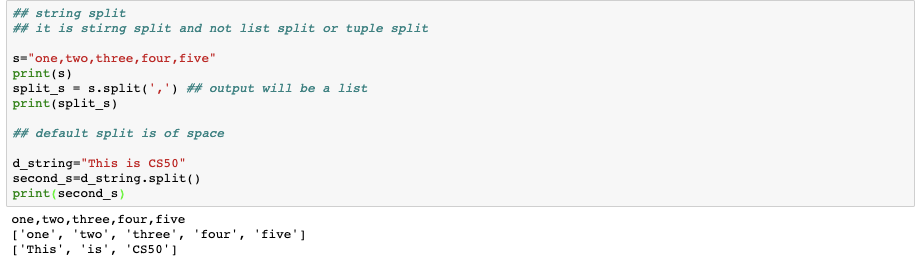
m - List Indexing :
- Each item in list has an assigned index value starting from 0.
- Accessing element in a list is called indexing .
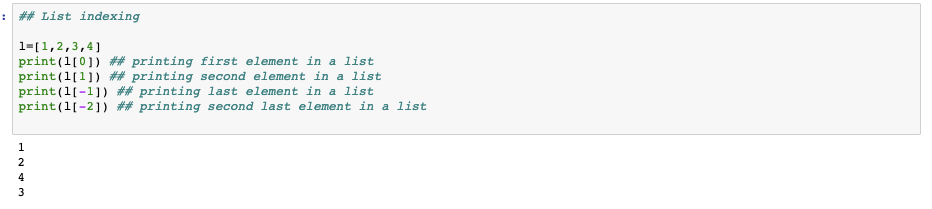
n - List Slicing :
- Accessing part of segments is called slicing .
- end value represents that the first value that is not present in the selected slice
eg : numbers[start:end:step_size].

o - List extend using + :

p - List Count :
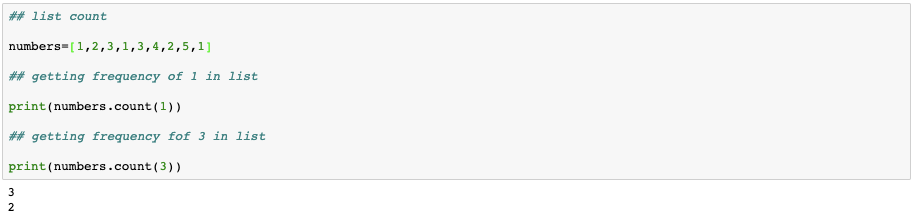
q - List Looping :
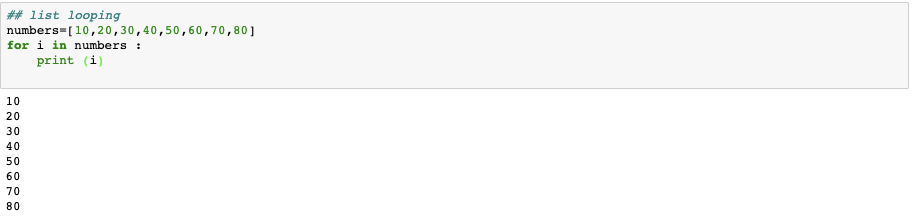
r - List Comprehensions :
- Provides concise way to create lists.
- Common applications : are to make new list where each element is result of some operation applied to each member of another sequence or iterable or to create a sequence of those elements to satisfy a certain condition .
eg:
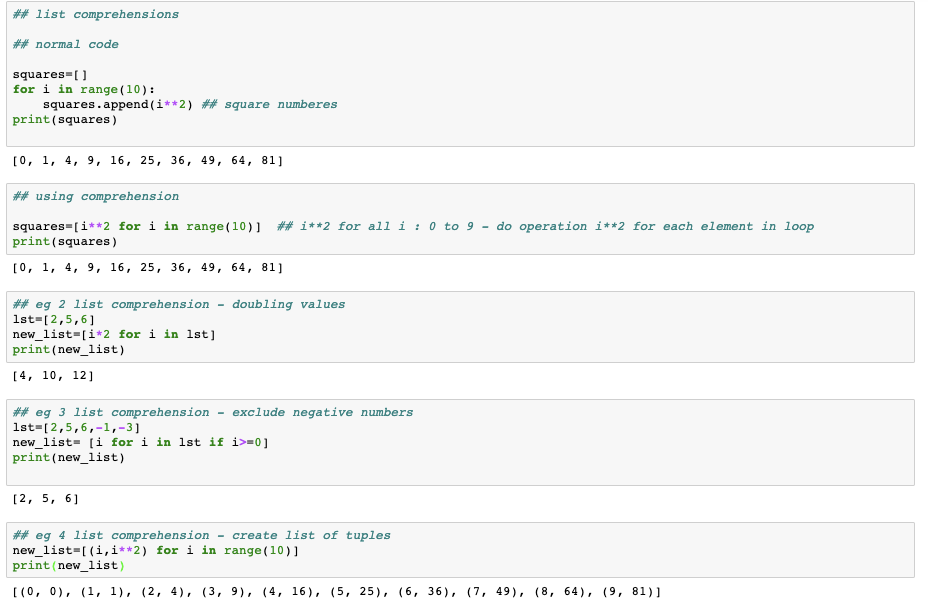
s - Nested List Comprehensions :
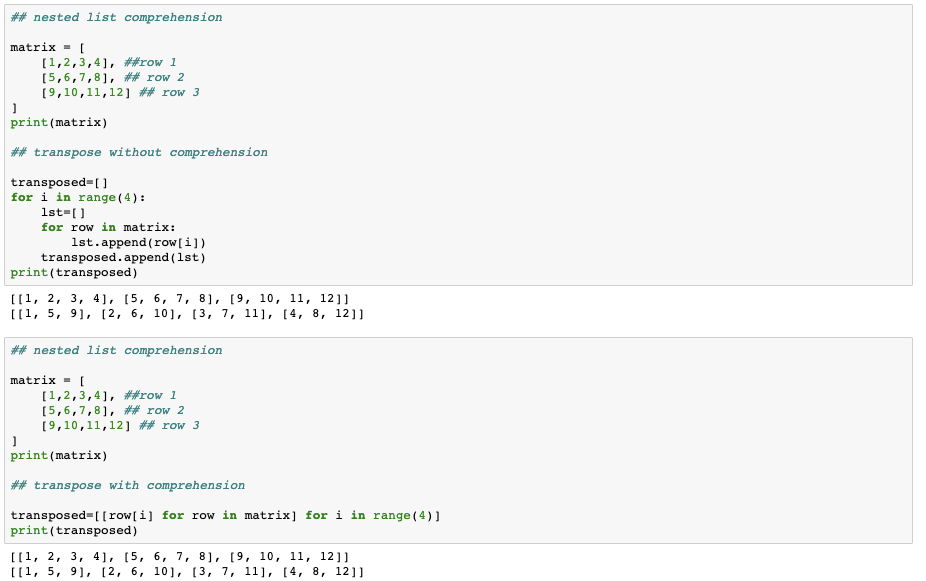
2 - Tuples
It is similar to list and enclosed in () .
Tuples are immutable ie elements cannot be changed or values cannot be updated.
Tuple operations :
a - Tuple Creation :
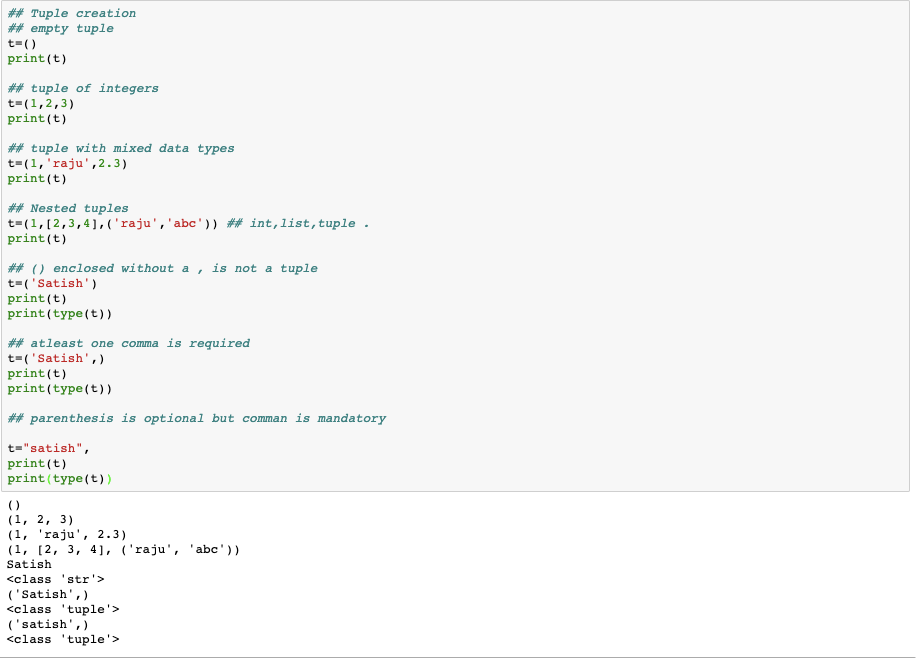
b - Accessing elements in Tuple creation :
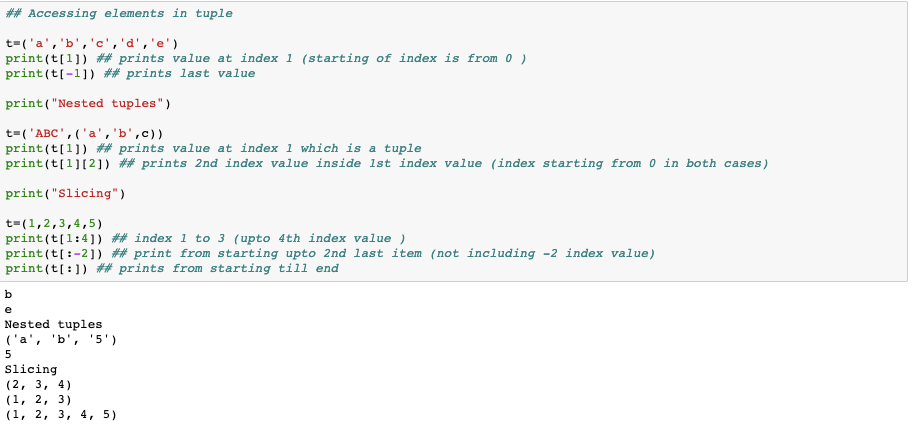
c - Changing a Tuple :
- Tuples are immutable.
- If element is itself of mutable datatype like list , it's nested items can be changed.
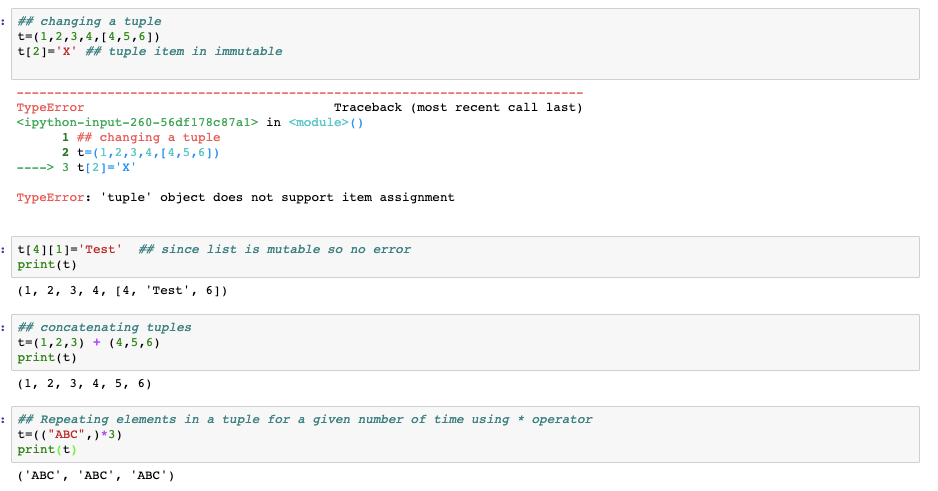
d - Tuple Deletion :
We cannot update/delete elements/items from a tuple but we can delete/remove entire tuple.
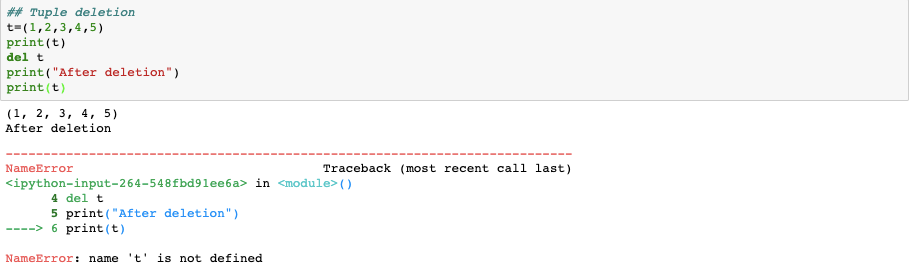
e - Tuple Count :

f - Tuple Index :

g - Tuple Membership :
Tests if value exists or not and returns True/False.

h - Tuple Length :

i - Tuple Sort :
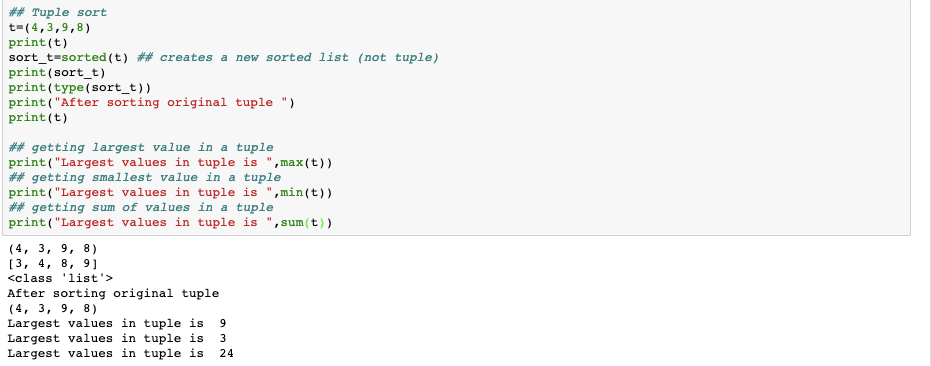
3 - Sets
They are unordered collection of items.
Every item is unique(no duplicates).
Sets are mutable ie we can add/remove items from it .
Cannot be indexed as not ordered.
Mathematical operations like union , intersection etc can be performed.
Sets operations :
a - Set Creation :
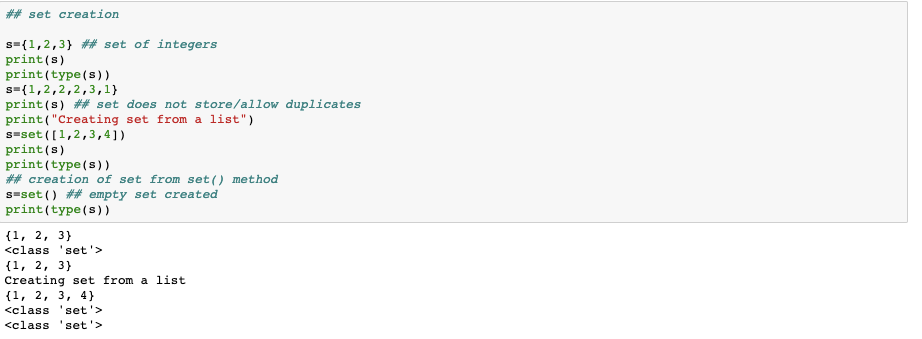
b - Add elements to Set :
- We can add single element using add() method and multiple elements via update(). method.

c - Remove elements from Set :
- methods :- remove(),discard(),pop(),clear.
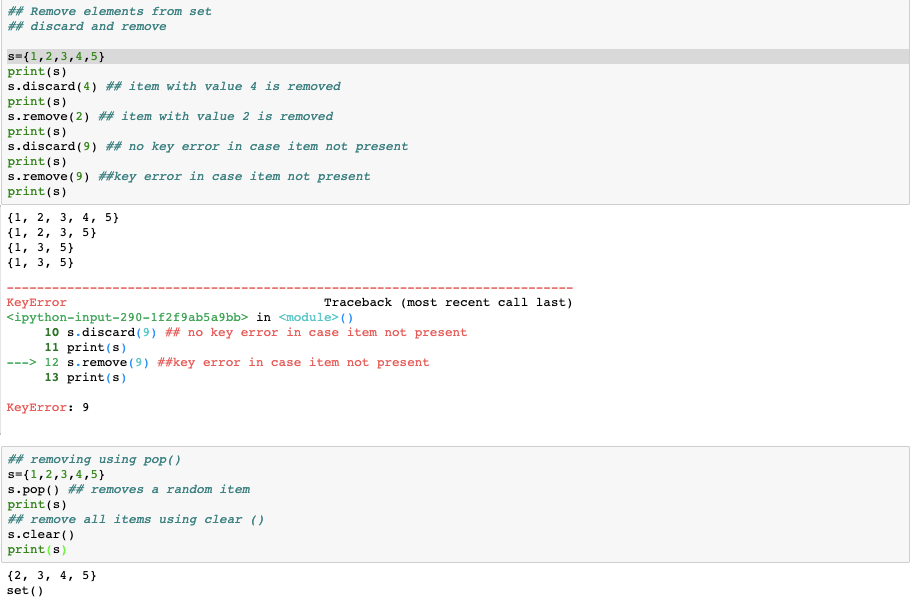
d - Mathematical Set operations:
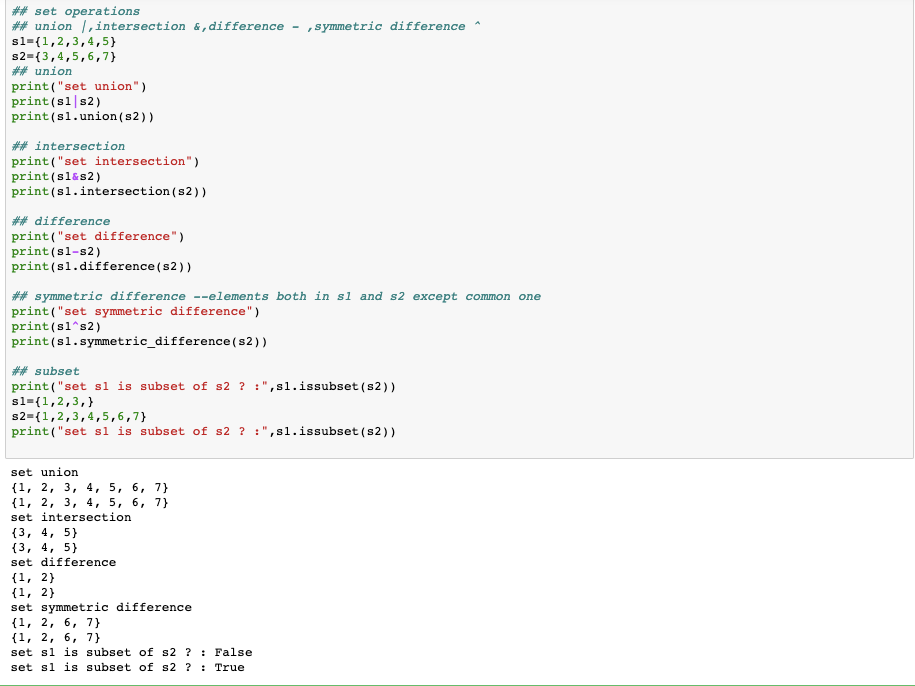
Frozen Sets :
- They have characteristics of set , but cannot be changed once assigned.
- Frozen sets are immutable sets.
- They are hash-able & can be used as keys to dictionary (normal sets cannot).
- Methods - copy() , difference(),intersection(), isdisjoint(), issubset(), issuperset(), symmetric_difference() & union().
- Does not have a method to add/remove elements since it is immutable.
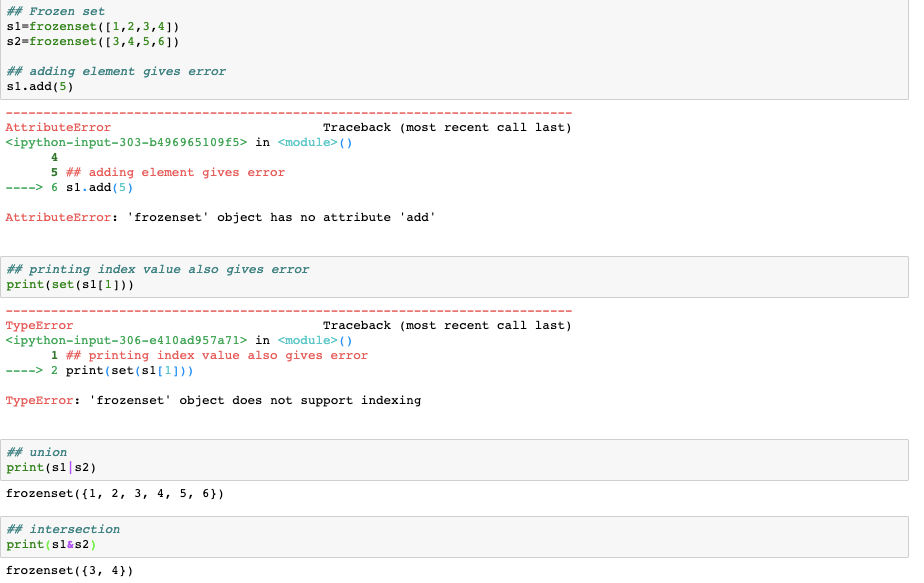
4 - Dictionary
It is unordered collection of items.
Cannot be indexed.
Dictionary has key:Value pair .
Dictionary operations :
a - Dictionary Creation :
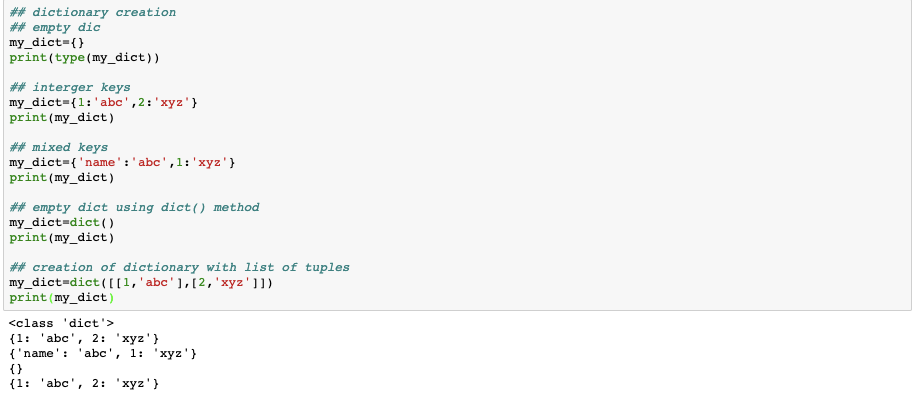
b - Dictionary Access :
- Internally hash function is used so access is very fast.
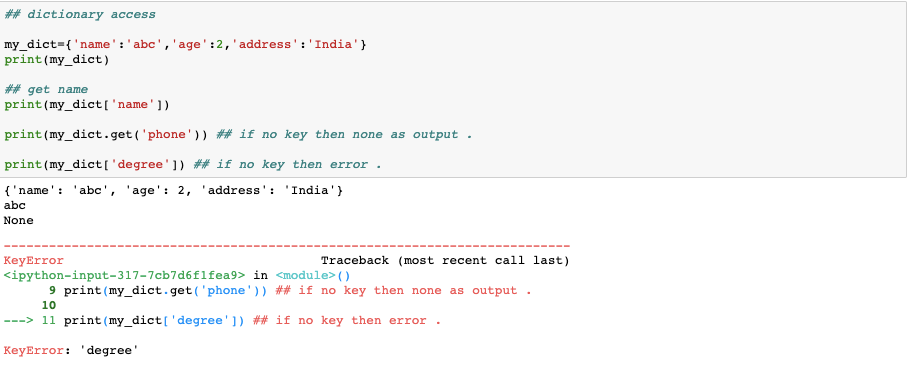
c - Dictionary Add/Modify :
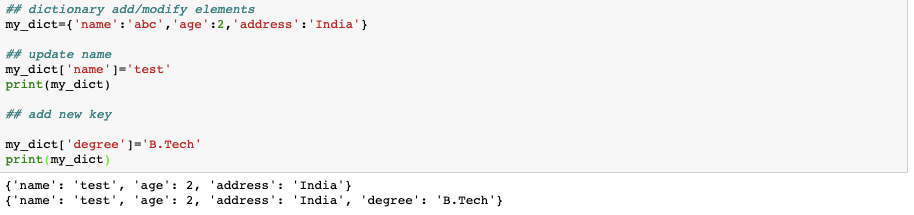
d - Dictionary Delete/Remove :
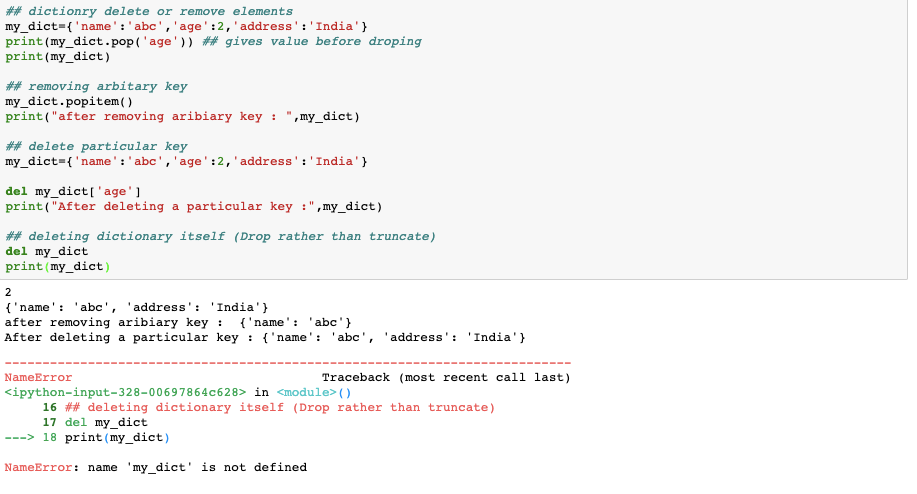
e - Dictionary Methods :
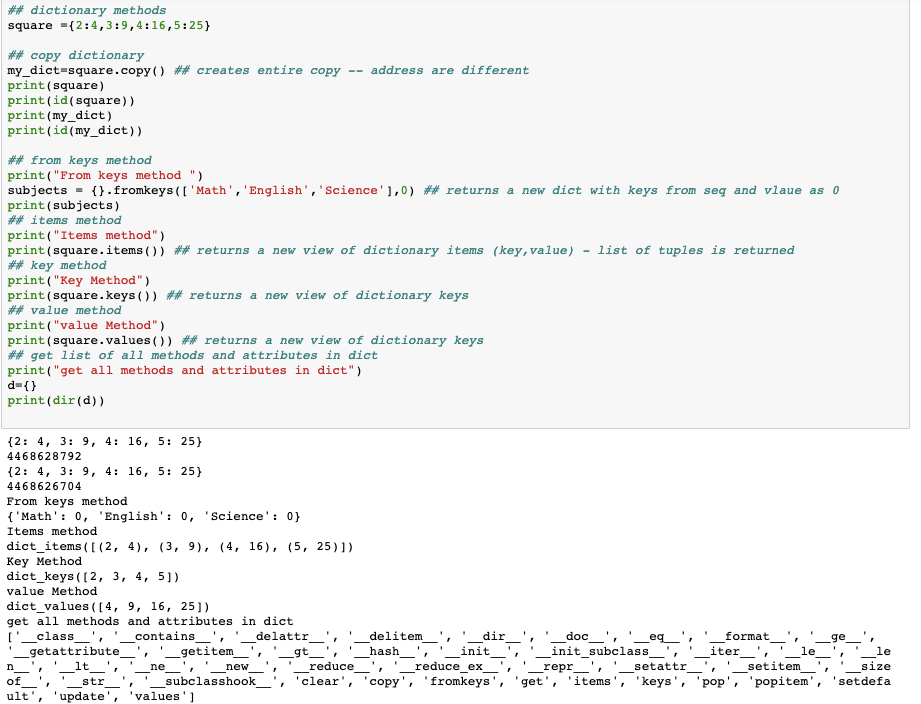
f - Dictionary Comprehension :
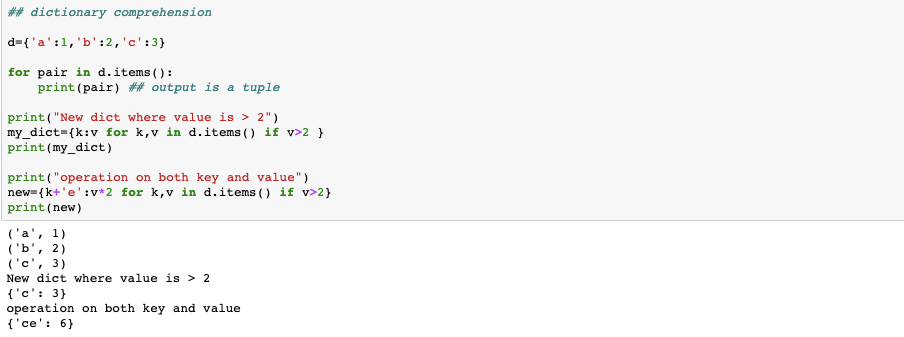
5 - Strings
String is a sequence of characters.
Computers don't understand characters they understand only binary numbers (Internally char is manipulated as 0's and 1's).
Conversion of a char to 0's and 1's is call encoding and reverse is decoding.
In Python string is a sequence of unicode characters (utf-8).
String operations :
a - String Creation :
- Single quotes, double quotes , triple quotes(generally used to represent multi-line string , docStrings and comments).
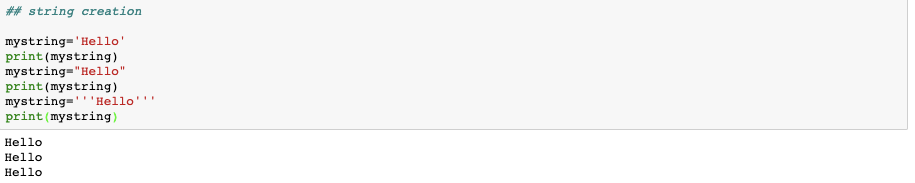
b - String Access :
- Using index access it .
- Index starts from 0.
- If out of index then error.
- Index must be integer.
- Negative indexes are allowed.

c - String Delete/change :
- They are immutable .
- Elements cannot be changed.
- We can reassign different strings to same name.
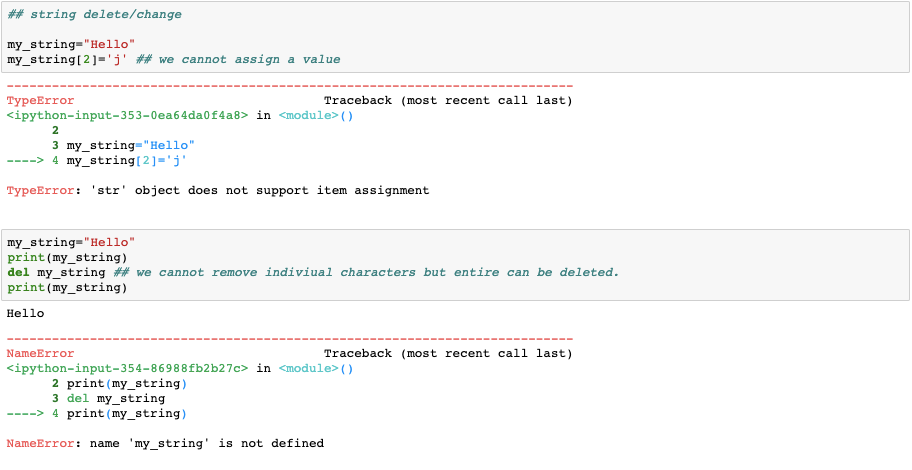
d - String Operations :

e - String Iteration :

f - String Membership :

g - String Methods :
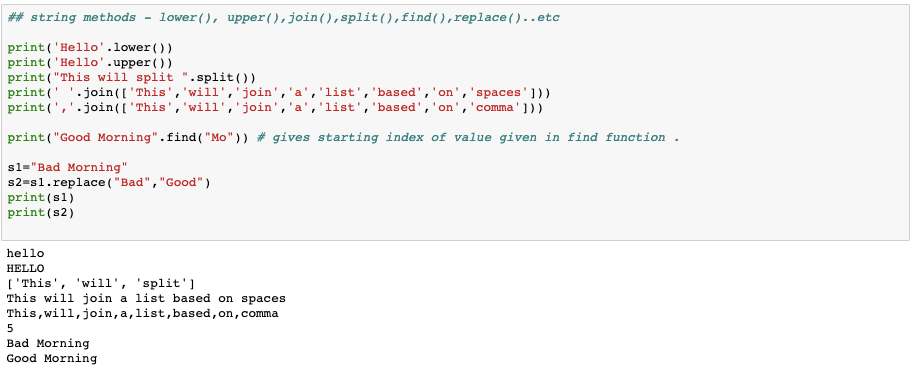
Comments